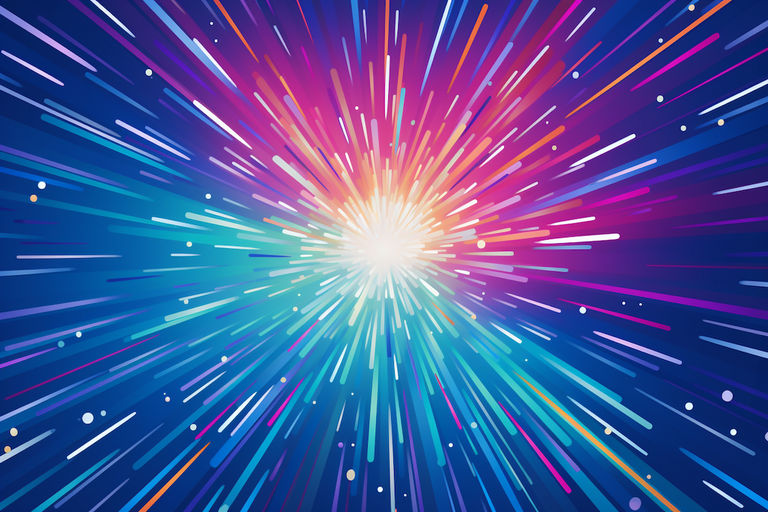
Speedier TypeScript Compilations with skipLibCheck
Hello, fellow developers! As someone who has been working with TypeScript for several years, I’ve come to appreciate the robust type-checking system it provides. However, I’ve also encountered situations where the compilation speed became a bottleneck, especially in large projects with numerous dependencies. Today, I want to share a practical tip that has helped me significantly improve compilation times: the skipLibCheck
option in the TypeScript configuration.
Understanding skipLibCheck
Before we dive into the details, let’s clarify what skipLibCheck
does. In TypeScript, when you import a library, the compiler not only checks your code but also the type definitions (*.d.ts
files) of the imported libraries. This thorough checking ensures type safety across your entire codebase. However, it comes at the cost of increased compilation time, particularly when you have a large number of dependencies.
The skipLibCheck
option, when set to true
in your tsconfig.json
file, instructs the TypeScript compiler to skip type checking for the declaration files of your imported libraries. Here’s how you can enable it:
{
"compilerOptions": {
"skipLibCheck": true
}
}
The Impact on Compilation Speed
In my experience, enabling skipLibCheck
can lead to a noticeable improvement in compilation speed, especially for projects with a heavy reliance on third-party libraries. I’ve worked on codebases where the compilation time was reduced from several minutes to just a few seconds by utilizing this option.
It’s important to note that the extent of the performance gain depends on factors such as the number and size of the imported libraries, as well as the complexity of their type definitions. Nonetheless, I’ve consistently observed faster compilations across various projects when skipLibCheck
is enabled.
Trade-offs and Considerations
While skipLibCheck
can provide a significant speed boost, it’s crucial to understand the trade-offs involved. By skipping library type checks, you’re essentially trusting the accuracy of the type definitions provided by the libraries. If there are errors or inconsistencies in those type definitions, they may go unnoticed during compilation.
In my experience, the vast majority of well-maintained libraries have reliable type definitions. However, it’s still a good practice to be aware of the libraries you’re using and their reputation within the community. If you encounter type-related issues that seem to stem from a library, you may need to investigate further and potentially report the issue to the library maintainers.
When to Use skipLibCheck
Deciding whether to use skipLibCheck
depends on your specific project requirements and priorities. Here are some scenarios where I believe it can be particularly beneficial:
-
Large Codebases with Many Dependencies: If your project heavily relies on numerous third-party libraries, enabling
skipLibCheck
can significantly reduce compilation times, allowing for faster development iterations. -
Continuous Integration and Deployment (CI/CD) Pipelines: In CI/CD pipelines, faster compilation times can lead to quicker feedback loops and more efficient resource utilization. Enabling
skipLibCheck
can help optimize these pipelines. -
Rapid Prototyping and Experimentation: When you’re in the early stages of a project or exploring new ideas, faster compilations can facilitate quicker iterations and experimentation.
On the other hand, if your project has a strong emphasis on type safety and you have encountered issues related to library type definitions in the past, you may want to exercise caution when enabling skipLibCheck
. In such cases, the extra type checking might be worth the compilation time trade-off.
Practical Tips
Here are a few practical tips I’ve learned while working with skipLibCheck
:
-
Selective Enabling: You can selectively enable
skipLibCheck
for specific builds or configurations. For example, you might enable it for development builds to improve iteration speed but disable it for production builds to ensure comprehensive type checking. -
Gradual Adoption: If you’re unsure about enabling
skipLibCheck
across your entire project, consider starting with a subset of your codebase or a specific feature branch. Monitor the impact on compilation speed and any potential type-related issues that may arise. -
Regular Library Updates: Keep your project’s dependencies up to date. Library maintainers often release updates that include type definition improvements and bug fixes. Regularly updating your dependencies can help mitigate potential issues related to skipping library type checks.
Conclusion
The skipLibCheck
option in TypeScript’s configuration provides a practical way to speed up compilation times, especially in projects with numerous dependencies. By skipping type checking for library declaration files, you can significantly reduce compilation overhead and improve developer productivity.
However, it’s essential to weigh the trade-offs and consider your project’s specific requirements. While skipLibCheck
can be a valuable tool in many scenarios, it’s not a one-size-fits-all solution. Use it judiciously and in combination with other best practices to strike a balance between compilation speed and type safety.
I hope this guide has provided you with a practical understanding of skipLibCheck
and how it can benefit your TypeScript projects. As always, keep exploring, learning, and optimizing your development workflow. Happy coding! 🚀✨
Stay in touch
Don't miss out on new posts or project updates. Hit me up on X (Twitter) for updates, queries, or some good ol' tech talk.
Follow @zkMake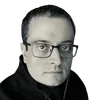