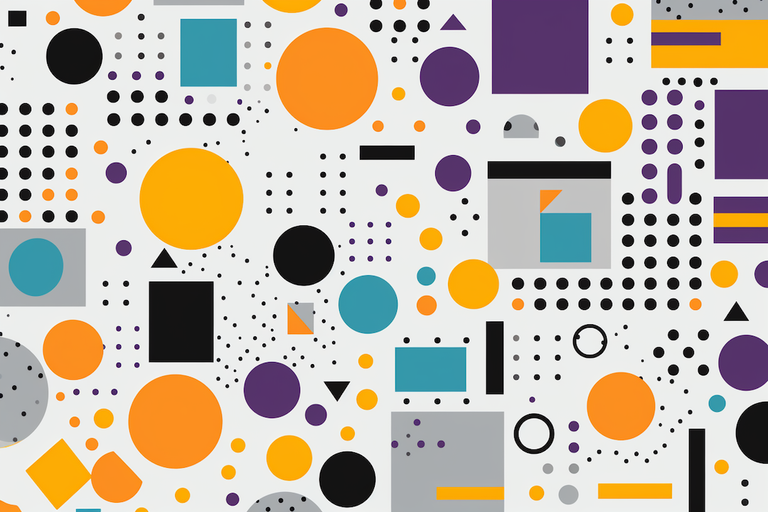
Name Game: Why You Should Favor Named Exports in ES Modules
As a software engineer having worked on large-scale JavaScript projects, I’ve witnessed firsthand the evolution of the language and its module system. The introduction of ECMAScript (ES) modules was a game-changer, bringing a standardized format to the chaotic world of JavaScript modules. However, with this new system came a common developer dilemma: should we use named exports or default exports? While both have their place, I’ve found compelling reasons to favor named exports in most scenarios. In this comprehensive guide, I’ll share my insights and experiences to help you make informed decisions when structuring your modules.
1. Crystal Clear Functionality with Named Exports
One of the most significant advantages of named exports is the transparency they bring to your codebase. When you open a module file, you can immediately identify what functionalities are being exported without having to read through the entire module. It’s like having a clear table of contents in a well-structured book.
Consider the following example:
// utils.js
export const add = (a, b) => a + b;
export const subtract = (a, b) => a - b;
export const multiply = (a, b) => a * b;
export const divide = (a, b) => a / b;
With named exports, anyone reading the utils.js
module can instantly understand that it provides basic arithmetic functions: add
, subtract
, multiply
, and divide
. This clarity is particularly valuable when working with larger modules that export multiple functionalities.
2. Enforcing Consistency Across the Codebase
In a real-world project, multiple developers often work on the same codebase, each bringing their own coding styles and preferences. Named exports help enforce a level of consistency by requiring specific identifiers for each exported entity. When different developers import and use the same functionality, they reference it by the same name, reducing confusion and potential naming conflicts.
Let’s compare two approaches:
// Without named exports
import MainComponent from "./Component";
import mainComponent from "./Component";
import MAIN_COMPONENT from "./Component";
// With named exports
import { MainComponent } from "./Component";
import { MainComponent } from "./Component";
import { MainComponent } from "./Component";
With named exports, the imported component is always referenced as MainComponent
, regardless of the developer’s individual preferences. This consistency becomes increasingly important as the project grows and more developers collaborate on the same codebase.
3. Simplified Refactoring with Modern Tooling
Refactoring is an integral part of any software development process. As your project evolves, you may need to rename certain exports to better reflect their functionality or to adhere to updated naming conventions. With named exports, modern tooling like ESLint or TypeScript can significantly simplify the refactoring process.
For example, let’s say you have a named export fetchUserData
that you want to rename to fetchUser
to be more concise. With the help of tools like ESLint, you can automatically update all the import statements across your codebase that reference fetchUserData
. This ensures that the renamed export is consistently reflected in all the files without requiring manual intervention.
// Before refactoring
import { fetchUserData } from "./api";
// After refactoring
import { fetchUser } from "./api";
This automated refactoring process saves a tremendous amount of time and effort, especially in larger codebases with numerous imports.
4. Granular Control with Selective Imports
Named exports empower developers with granular control over what they import from a module. Instead of importing the entire module, you can selectively choose the specific functions, variables, or classes that you need. This not only improves code efficiency but also makes the intent of your code more explicit.
Consider the following example:
// Importing the entire module
import * as MathUtils from "./mathUtils";
// Selectively importing specific named exports
import { calculateAverage, generateRandomNumber } from "./mathUtils";
By selectively importing calculateAverage
and generateRandomNumber
, you make it clear that your code only relies on those specific functionalities from the mathUtils
module. This clarity enhances code readability and maintainability, especially for other developers who may work on the same codebase in the future.
5. Enhanced Developer Experience with Autocomplete
As developers, we often find ourselves working with modules that export a wide range of functionalities. Remembering the exact names of all the exports can be challenging, especially when dealing with complex or unfamiliar codebases. This is where named exports truly shine.
Modern code editors and integrated development environments (IDEs) provide intelligent autocompletion capabilities based on the named exports of a module. When you start typing the name of an import, the editor suggests the available named exports, allowing you to quickly select the desired functionality without having to refer back to the module file.
For instance, let’s say you have a module utils.js
with multiple named exports:
// utils.js
export const formatDate = (date) => {...};
export const parseJSON = (json) => {...};
export const generateUUID = () => {...};
// ... more named exports
When importing from this module, your code editor’s autocompletion feature will provide suggestions for formatDate
, parseJSON
, generateUUID
, and any other named exports available. This saves valuable time and reduces the cognitive load of remembering exact export names, allowing you to focus on writing meaningful code.
6. Clarity and Intent with Explicit Naming
One of the key benefits of named exports is the clarity and intent they bring to your code. By explicitly naming your exports, you provide a clear indication of what each export represents and how it should be used.
Let’s compare the use of default exports and named exports:
// With default export
import Util from "./utils";
// With named export
import { formatCurrency } from "./utils";
In the first example, the default export is imported as Util
, which doesn’t convey much information about what the imported functionality does. On the other hand, the named export formatCurrency
explicitly communicates its purpose, making the code more self-explanatory.
This explicitness becomes even more valuable when dealing with complex modules that export multiple functionalities. Named exports enforce a level of clarity and intent, reducing ambiguity and making the code more maintainable in the long run.
7. Optimized Bundles with Tree Shaking
In modern JavaScript development, bundle optimization is crucial for building performant applications. One technique that has gained popularity is tree shaking, which eliminates unused code from the final bundle. While modern bundlers like webpack and Rollup can tree-shake both named and default exports, named exports make the process more reliable and effective.
When you use named exports, the bundler can easily identify which exports are actually being used in your codebase and include only those in the final bundle. This results in smaller bundle sizes and faster load times for your application.
Consider the following example:
// math.js
export const add = (a, b) => a + b;
export const subtract = (a, b) => a - b;
export const multiply = (a, b) => a * b;
export const divide = (a, b) => a / b;
// main.js
import { add, multiply } from "./math";
console.log(add(2, 3));
console.log(multiply(4, 5));
In this case, the bundler can safely eliminate the unused subtract
and divide
exports from the final bundle, reducing its size and improving performance.
8. Flexibility for Multifunctional Modules
In real-world projects, modules often serve multiple purposes and export various functionalities. Named exports provide the flexibility to handle such multifunctional modules without any constraints.
Let’s consider a module that handles data fetching and manipulation:
// dataUtils.js
export const fetchData = async (url) => {
const response = await fetch(url);
return response.json();
};
export const transformData = (data) => {
// Perform data transformation
return transformedData;
};
export const filterData = (data, criteria) => {
// Filter data based on criteria
return filteredData;
};
With named exports, you can easily export multiple functionalities from the same module, each serving a specific purpose. This allows for a more modular and reusable codebase, as different parts of your application can import and use only the functionalities they need.
9. Consistent Naming Conventions
One potential pitfall of default exports is the lack of naming consistency across different files. Since default exports can be imported with any name, different developers might use varying names for the same imported functionality, leading to confusion and inconsistencies.
Named exports, on the other hand, enforce a consistent naming convention. The imported name must match the exported name, ensuring that all developers use the same identifier for a particular functionality.
// Component.js
export const DataComponent = () => {...};
// Developer A
import { DataComponent } from "./Component";
// Developer B
import { DataComponent } from "./Component";
In this example, both Developer A and Developer B are required to use the DataComponent
identifier when importing from the Component.js
module. This consistency makes the codebase more predictable and easier to understand, especially when multiple developers are collaborating on the same project.
Conclusion
In my years of experience as a software engineer, I’ve found that favoring named exports in ES modules brings numerous benefits to the development process. From improved code clarity and consistency to simplified refactoring and optimized bundles, named exports provide a solid foundation for building maintainable and scalable JavaScript applications.
While default exports have their place, particularly for modules with a single primary export, named exports offer a more explicit and flexible approach. They promote transparency, encourage modular design, and enhance the overall developer experience.
As you tackle your next JavaScript project or refactor an existing codebase, I highly recommend embracing named exports. The clarity, consistency, and tooling support they provide will pay dividends in the long run, making your code more readable, maintainable, and collaborative.
Remember, the choices you make in structuring your modules have a significant impact on the overall health and longevity of your codebase. By favoring named exports, you’re setting yourself up for success and paving the way for a more robust and efficient development process.
Stay in touch
Don't miss out on new posts or project updates. Hit me up on X (Twitter) for updates, queries, or some good ol' tech talk.
Follow @zkMake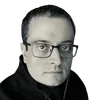