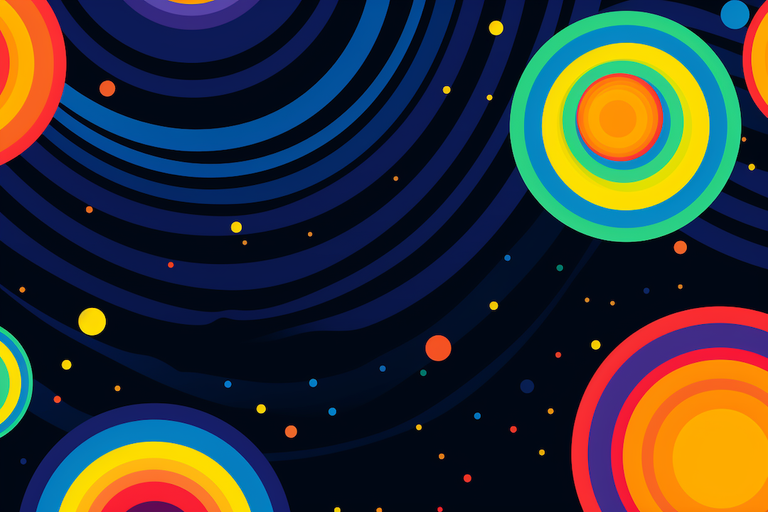
JavaScript Module Systems Unraveled: Which One to Use and When
As a frontend software engineer, I’ve had my fair share of experiences working with different JavaScript module systems. Today, I want to share some insights and practical advice to help you navigate the sometimes confusing world of JavaScript modules. Whether you’re a beginner trying to make sense of it all or an experienced developer looking to refine your understanding, this guide will shed light on the various module systems, their use cases, and best practices.
Understanding the Need for Modules
Before we explore the different module systems, it’s crucial to understand why we need modules in the first place. As JavaScript applications grow in size and complexity, managing code becomes increasingly challenging. Modules allow us to break down our codebase into smaller, reusable, and maintainable chunks. By encapsulating related functionality into separate files, we can achieve better code organization, avoid naming conflicts, and promote code reusability.
ESM: The Modern Standard
ECMAScript Modules (ESM) is the modern standard for working with modules in JavaScript. Introduced in ES6 (ES2015), ESM provides a native and standardized way to define and consume modules. With ESM, you can use the export
keyword to expose specific functions, variables, or classes from a module, and the import
keyword to bring them into another file.
Here’s a practical example of ESM in action:
// math.js
export function add(a, b) {
return a + b;
}
export function subtract(a, b) {
return a - b;
}
// main.js
import { add, subtract } from "./math.js";
console.log(add(5, 3)); // Output: 8
console.log(subtract(10, 4)); // Output: 6
ESM is supported in all modern browsers and Node.js versions (starting from v12), making it the preferred choice for new projects. It offers features like static analysis, tree shaking, and efficient dead-code elimination, resulting in smaller bundle sizes and improved performance.
CommonJS: The Node.js Way
CommonJS is the module system that has been widely used in Node.js since its early days. It follows a synchronous approach, where modules are loaded and executed in a blocking manner. In CommonJS, you use the require
function to import a module and the exports
object to export functionality.
Here’s an example of CommonJS:
// utils.js
exports.capitalize = function (str) {
return str.charAt(0).toUpperCase() + str.slice(1);
};
// main.js
const utils = require("./utils");
console.log(utils.capitalize("hello")); // Output: "Hello"
While CommonJS has been the de facto standard in the Node.js ecosystem, it has some limitations. It lacks native support in browsers, and its synchronous nature can lead to performance bottlenecks in certain scenarios. However, CommonJS is still widely used in server-side Node.js applications and in projects with a large existing codebase.
AMD and UMD: Bridging the Gap
Asynchronous Module Definition (AMD) and Universal Module Definition (UMD) are module systems that were developed to address the limitations of CommonJS in browser environments.
AMD, popularized by libraries like RequireJS, allows for asynchronous loading of modules, which is essential for optimizing browser performance. It uses the define
function to define modules and the require
function to load them asynchronously.
UMD, on the other hand, is a module system that aims to provide compatibility across different environments. It can work with both AMD and CommonJS, making it a good choice for libraries that need to support multiple module systems.
While AMD and UMD have their place in certain scenarios, with the widespread adoption of ESM and the availability of bundlers like webpack and Rollup, their usage has diminished in modern web development.
Best Practices and Tips
-
Use ESM whenever possible: If you’re starting a new project or working in a modern JavaScript environment, ESM should be your go-to choice. It offers a clean and standardized syntax, better performance, and wide browser support.
-
Stick to a single module system: Consistency is key when working with modules. Avoid mixing different module systems within the same project to prevent confusion and maintainability issues.
-
Use descriptive names for modules: Choose clear and descriptive names for your modules to make their purpose evident. This improves code readability and makes it easier for other developers to understand and navigate your codebase.
-
Keep modules focused and cohesive: Each module should have a single responsibility and encapsulate related functionality. Avoid creating monolithic modules that do too much. Smaller, focused modules are easier to understand, test, and maintain.
-
Leverage bundlers for optimization: When working with ESM in web projects, use bundlers like webpack, Rollup, or Parcel to optimize your code. These tools can handle module resolution, tree shaking, and code splitting, resulting in faster load times and smaller bundle sizes.
Embracing the Module Mindset
As you dive deeper into JavaScript development, embracing the module mindset becomes increasingly important. Modular code is not only easier to understand and maintain but also promotes code reuse and collaboration among team members.
Remember, the choice of module system depends on your project’s requirements, the environment you’re targeting, and the ecosystem you’re working with. By understanding the strengths and use cases of each module system, you can make informed decisions and write modular, maintainable JavaScript code.
Keep exploring, keep refining your skills, and most importantly, enjoy the process of crafting efficient and elegant JavaScript modules. Happy coding!
Stay in touch
Don't miss out on new posts or project updates. Hit me up on X (Twitter) for updates, queries, or some good ol' tech talk.
Follow @zkMake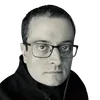