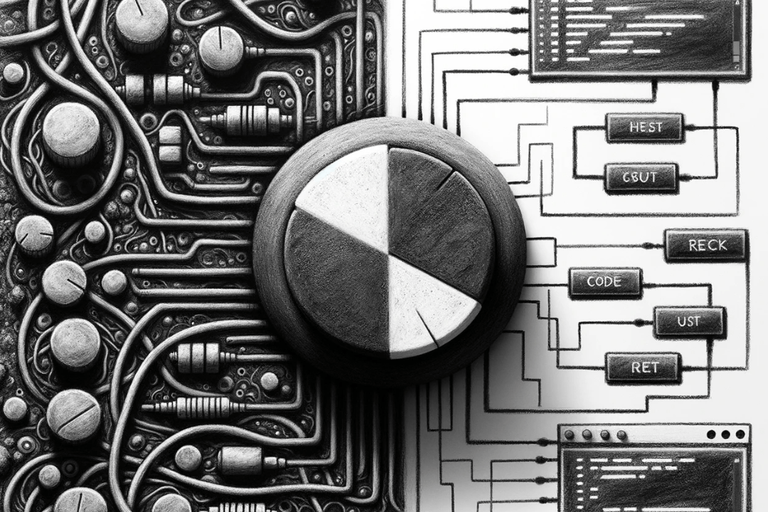
Making Signal Out of Noise: React as Your Ultimate UI Tuner
In the crowded frequency spectrum of programming, the concept of signal vs. noise is often overlooked. Yet, it’s the essence of why we develop abstraction layers, frameworks, and libraries. They all aim to extract the important ‘signal’ from the messy ‘noise’ that is inherent in any complex system. It’s time we discuss React in this context: as a tool that can turn the cacophony of frontend development into a harmonious melody.
The Universal Principle: Signal vs. Noise
Let’s refresh our understanding of signal and noise. Signal is the meaningful information or output you want to get from a system. When thinking about UI, it’s the user interactions, the animations, and the layout—essentially, the experience you’re aiming to deliver. Noise is everything else—the boilerplate, the repeated code, the unnecessary complications that make the signal harder to discern.
So, when we talk about React, it’s not just a library for building UIs—it’s a methodology for making signal out of noise.
The Component Architecture: Translating Noise into Signal
The whole notion of React’s component-based architecture is a case study in signal extraction. Take for instance, a bloated jQuery codebase where DOM manipulations are mixed with logic. Now reimagine this in React. You create self-contained components that make it clear what a section of the UI does.
// Button.tsx
import React from "react";
import clsx from "clsx";
import styles from "./Button.module.css";
type ButtonProps = {
label: string;
onClick: () => void;
variant?: "primary" | "secondary";
};
const Button: React.FC<ButtonProps> = ({
label,
onClick,
variant = "primary",
}) => {
const buttonClass = clsx(styles.button, {
[styles.primary]: variant === "primary",
[styles.secondary]: variant === "secondary",
});
return (
<button className={buttonClass} onClick={onClick}>
{label}
</button>
);
};
export default Button;
The noise (manipulating the DOM, attaching event listeners, etc.) is abstracted away. What’s left? A ‘Button’ component that is the very definition of signal—it performs a specific action, and its props define how it looks and behaves.
CSS: The Background Noise
Let’s face it—CSS can be a noisy mess. But guess what? React lends its signal-making prowess to CSS as well. With techniques like CSS-in-JS or even just modular CSS, you can tie your styles closely to your components.
/* Button.module.css */
.button {
cursor: pointer;
border: none;
padding: 12px 24px;
font-size: 16px;
}
.primary {
background-color: blue;
color: white;
}
.secondary {
background-color: grey;
color: black;
}
Now, your CSS is not just a bunch of global styles. It’s segmented and co-located with your React components, reducing the noise and elevating the signal.
TypeScript as a Signal Amplifier
If React is your signal tuner, TypeScript is the amplifier that makes that signal loud and clear. By adding static types to JavaScript, TypeScript enhances the already strong abstraction layer that React provides. Consider a simple user profile display:
type UserProfileProps = {
user: {
id: string;
name: string;
age: number;
email: string;
};
};
const UserProfile = (props: UserProfileProps) => {
const { user } = props;
return <div>{/* User profile UI here */}</div>;
};
TypeScript eliminates the noise of runtime errors, bad property accesses, and undefined variables. Your components are clean, your props are self-documenting, and the signal comes through loud and clear.
React’s Ecosystem: The Signal Processing Suite
Let’s not forget the robust ecosystem of libraries and tools that surround React—Redux for state management, Jest for testing, and many more. These tools further refine the signal by taking away the repetitive, boilerplate noise, allowing you to focus on what truly matters: delivering an exceptional user experience.
Conclusion: The Engineering Behind Clarity
When it comes to frontend development, our aim is often to bring order to chaos. React, complemented by TypeScript and CSS practices, serves as a structured framework that helps us achieve this goal. It’s not just about compartmentalizing code or making it ‘look clean.’ It’s about creating a codebase that prioritizes the essential elements—your UI components, business logic, and user interactions—while reducing the distractions and pitfalls that can lead to errors and subpar user experiences.
By embracing React as a means of distilling signal from noise, you’re not just following best practices; you’re enhancing the clarity, reliability, and maintainability of your applications. And in a field often marked by complexity and rapid change, these are qualities that set apart good software from great software.
Stay in touch
Don't miss out on new posts or project updates. Hit me up on X (Twitter) for updates, queries, or some good ol' tech talk.
Follow @zkMake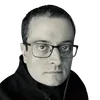