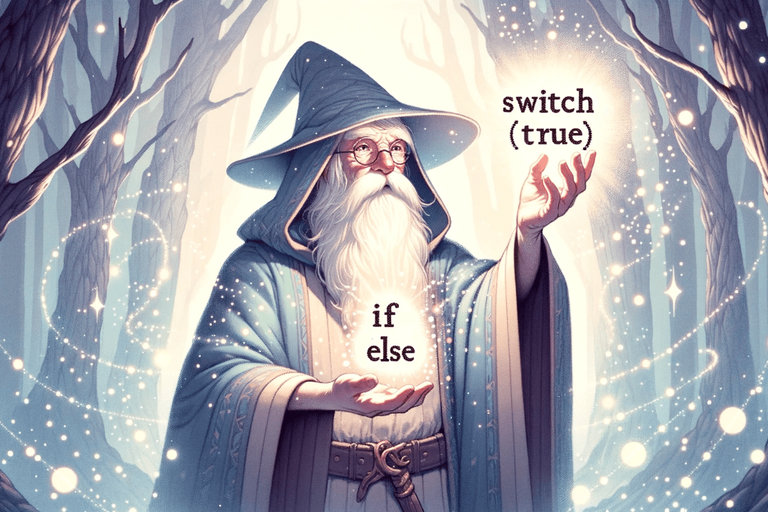
switch(true): The Stylish Way to Simplify Complex Conditionals in React
Hey there, fellow code wranglers! 🚀
As a frontend React engineer, I’ve encountered my fair share of conditional rendering challenges in React. Whether it’s crafting intricate if-else
chains or wrestling with nested switch-case
statements, we’ve all been there. But recently, I stumbled upon a lesser-known technique that has revolutionized the way I approach complex conditionals: switch(true)
.
Now, I know what you might be thinking. “Wait, what? switch(true)
? That sounds like some sort of coding voodoo! 🪄” Trust me, I had the same reaction when I first discovered this technique. It’s not something you typically learn in coding bootcamps or beginner tutorials. But once I started using it in my projects, I realized just how powerful and expressive it could be.
The Usual Suspects: If-Else Ladders and Switch-Case Blocks
Before we dive into the magic of switch(true)
, let’s take a quick look at the conventional approaches to handling conditionals in React.
The If-Else Ladder 🪜
const Notification = ({ type }: { type: string }) => {
if (type === "success") {
return <SuccessNotification />;
} else if (type === "error") {
return <ErrorNotification />;
} else if (type === "warning") {
return <WarningNotification />;
} else {
return <DefaultNotification />;
}
};
We’ve all written code like this at some point. It gets the job done, but as the number of conditions grows, the readability starts to suffer. It’s easy to get lost in a sea of curly braces and indentation.
The Classic Switch-Case 🎰
const Notification = ({ type }: { type: string }) => {
switch (type) {
case "success":
return <SuccessNotification />;
case "error":
return <ErrorNotification />;
case "warning":
return <WarningNotification />;
default:
return <DefaultNotification />;
}
};
The switch-case
block is a tried-and-true solution for handling multiple conditions. It’s more concise than an if-else
ladder, but it can still become unwieldy when dealing with complex logic or multiple variables.
Introducing switch(true): The Unconventional Hero 🦸♂️
This is where switch(true)
comes in to save the day. It’s a bit of an oddball because it goes against the traditional usage of switch
statements. Instead of matching a value against different cases, switch(true)
evaluates expressions within each case
statement.
switch(true) in Action 🎬
const Notification = ({ type }: { type: string }) => {
switch (true) {
case type === "success":
return <SuccessNotification />;
case type === "error":
return <ErrorNotification />;
case type === "warning":
return <WarningNotification />;
default:
return <DefaultNotification />;
}
};
Voila! 🪄 The code becomes much more readable and expressive. Each case
statement directly evaluates a condition, making it crystal clear what each branch represents.
Unleashing the Full Potential of switch(true) 🚀
Now that we’ve seen the basic usage of switch(true)
, let’s explore some more advanced techniques to make the most of this approach.
Combining Multiple Conditions 🧩
One of the strengths of switch(true)
is its ability to handle multiple conditions elegantly. Check out this example:
const UserInfo = ({
status,
isAdmin,
}: {
status: string;
isAdmin: boolean;
}) => {
switch (true) {
case status === "active" && isAdmin:
return <AdminActiveUser />;
case status === "inactive" && isAdmin:
return <AdminInactiveUser />;
case status === "active":
return <ActiveUser />;
default:
return <InactiveUser />;
}
};
By using switch(true)
, we can easily combine multiple conditions within each case
statement. This allows us to handle complex scenarios without resorting to deeply nested if-else
blocks.
Leveraging Functions and Ternary Operators ⚙️
We can take things a step further by incorporating functions and ternary operators within our switch(true)
statements:
const isMajorVersionUpdate = (currentVersion: string, newVersion: string) => {
return parseInt(newVersion, 10) > parseInt(currentVersion, 10);
};
const UpdateNotification = ({
currentVersion,
newVersion,
}: {
currentVersion: string;
newVersion: string;
}) => {
switch (true) {
case isMajorVersionUpdate(currentVersion, newVersion):
return <MajorVersionNotification />;
case newVersion !== currentVersion:
return <MinorVersionNotification />;
default:
return <NoUpdateNotification />;
}
};
By extracting complex logic into separate functions, we can keep our switch(true)
block clean and focused. This also makes it easier to unit test our code, as we can test the individual functions in isolation.
Real-World Examples and Best Practices 🌍
Over the years, I’ve found switch(true)
to be particularly useful in scenarios where I need to handle multiple conditions based on different variables or complex expressions. Here are a few real-world examples where switch(true)
has proven its worth:
- User Permissions: When building user interfaces, we often need to conditionally render components based on the user’s role or permissions.
switch(true)
allows us to cleanly express these conditions and keep our code readable.
const UserDashboard = ({ user }: { user: User }) => {
switch (true) {
case user.isAdmin:
return <AdminDashboard />;
case user.isManager:
return <ManagerDashboard />;
case user.isEmployee:
return <EmployeeDashboard />;
default:
return <GuestDashboard />;
}
};
- API Response Handling: When working with API responses, we often need to conditionally render different components based on the response status or data.
switch(true)
can help us handle these scenarios elegantly.
const ApiResponseHandler = ({ response }: { response: ApiResponse }) => {
switch (true) {
case response.status === 200 && response.data:
return <SuccessComponent data={response.data} />;
case response.status === 404:
return <NotFoundComponent />;
case response.status >= 400 && response.status < 500:
return <ClientErrorComponent />;
case response.status >= 500:
return <ServerErrorComponent />;
default:
return <LoadingComponent />;
}
};
- Form Validation: When building forms, we often need to display different error messages or perform different actions based on the validation state.
switch(true)
can help us express these conditions clearly.
const FormSubmitHandler = ({ formState }: { formState: FormState }) => {
switch (true) {
case formState.isValid && formState.isDirty:
return <SubmitButton />;
case !formState.isValid && formState.isDirty:
return <ErrorMessage />;
default:
return <DisabledSubmitButton />;
}
};
Of course, as with any coding technique, it’s important to use switch(true)
judiciously. If you find yourself writing overly complex expressions within each case
statement, it might be a sign that you need to refactor your code or break things down into smaller, more manageable functions.
Wrapping Up 🎁
Well, there you have it, folks! switch(true)
is a powerful tool that can help you tame complex conditionals in your React components. It’s not a silver bullet, but when used appropriately, it can make your code more expressive, readable, and maintainable.
As with any coding pattern or technique, it’s important to consider the trade-offs and to use switch(true)
in a way that aligns with your team’s coding standards and best practices. Some developers might find it a bit unconventional at first, but I encourage you to give it a try and see how it can simplify your conditional rendering logic.
Remember, the goal is not to use switch(true)
everywhere just because it’s a cool trick. Instead, use it strategically in scenarios where it genuinely improves the clarity and maintainability of your code.
I hope this deep dive into switch(true)
has been helpful and has given you a new tool to add to your React development arsenal. If you have any questions or want to share your own experiences with switch(true)
, feel free to reach out. I’m always excited to learn from fellow developers and exchange ideas.
Until next time, happy coding, and may your conditionals be forever readable! 🚀✨
Stay in touch
Don't miss out on new posts or project updates. Hit me up on X (Twitter) for updates, queries, or some good ol' tech talk.
Follow @zkMake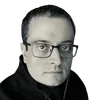